Integrating CodeSignal and React: A Detailed Overview
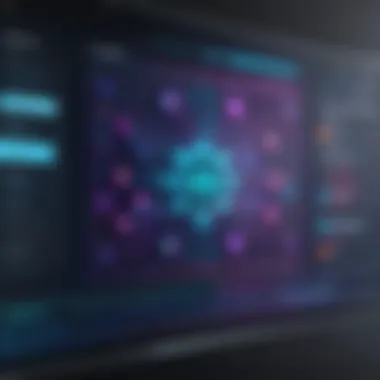
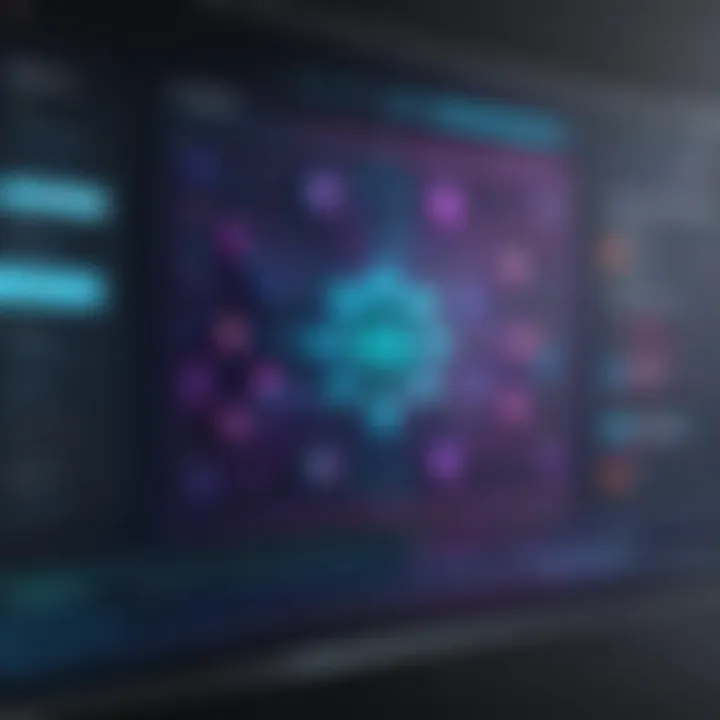
Intro
In the age of rapid software development, having the right tools in your toolkit can make all the difference. Among emerging platforms that assess coding skills, CodeSignal is becoming increasingly relevant, especially when paired with the powerful React library. This two-pronged approach not only streamlines the development process but also enhances the overall assessment of coding proficiency.
When you combine CodeSignal's capabilities with React, the functionality multiplies. This integration allows developers to create smooth user interfaces, while also implementing robust coding evaluations. The outcome? A well-rounded application that showcases both technical skills and user engagement. In this guide, we probe into how these two systems can work together, while also exploring their core features and competitive landscapes.
Understanding CodeSignal
In the fast-paced realm of software development, having the right tools can make a world of difference. CodeSignal stands as a pivotal platform in this landscape, particularly when it’s intertwined with React—a toolkit that's become ubiquitous among developers. It’s not just about writing code; it’s about writing code effectively. Understanding CodeSignal is crucial for any professional looking to enhance their development process, whether you're a seasoned coder or just diving into the world of programming.
Overview of CodeSignal
CodeSignal serves as a comprehensive assessment platform designed primarily for gauging coding skills. Unlike traditional methods, it leverages real-world challenges that developers face daily. Imagine being evaluated not just on textbook problems, but on scenarios you might actually encounter in the field. This approach makes it immensely valuable for coding assessments during hiring processes.
The interface is user-friendly and designed to simulate environments that developers are familiar with. From basic algorithms to complex system designs, it offers a variety of challenges. It’s not merely about passing a test; it’s about demonstrating your ability to navigate through coding intricacies in practical situations.
Importance in Software Development
The significance of CodeSignal in software development extends well beyond the individual user's experience. For businesses, leveraging CodeSignal during the hiring phase can lead to a better match between candidates and the roles they are filling. Data-driven insights provided by the platform assure employers that they are making informed decisions based on actual coding skills, rather than just resumes or interviews.
Moreover, utilizing CodeSignal promotes a culture of continuous learning within organizations. Setting up internal assessments can encourage developers to sharpen their coding skills regularly. Exposure to diverse coding challenges allows developers to think critically and creatively, which ultimately leads to higher quality software development.
Features and Capabilities
CodeSignal is loaded with features that cater to various needs within the development community. Here are some key capabilities:
- Variety of Assessments: Tests range from simple coding tasks to complex algorithmic challenges, ensuring a holistic evaluation of a candidate’s programming capability.
- Customizable Challenges: Organizations can tailor tests to suit specific needs, making it easier to find the right fit for particular roles.
- Real-time Collaboration Tools: Integrated coding environments allow interviewers to engage with candidates live, simulating real-world coding scenarios.
- Analytics Dashboard: The platform provides powerful analytics, which allow businesses to track progress and identify skill gaps among their teams.
"In a world where coding is king, having a reliable assessment tool like CodeSignal can set a candidate apart from the rest."
These features not only streamline the hiring process but also foster a deeper understanding of the skills necessary for success in various software development roles. This level of engagement leads to more productive teams and ultimately affects the success of projects.
By grasping the nuances of CodeSignal, organizations and developers can better navigate the complexities of modern software development, ensuring that they remain competitive in an ever-evolving industry.
Prologue to React
In this ever-evolving world of web development, understanding how React fits into the bigger picture is crucial. React is not merely a library; it’s a powerful tool that helps developers create dynamic user interfaces with ease. By integrating CodeSignal with React, developers can harness the advantages of both worlds—assessing coding skills while building interactive applications.
Why should developers pay attention to React? One key reason is React’s component-based architecture. It encourages code reuse, resulting in cleaner and maintainable code. This architecture is particularly beneficial in larger applications where managing the user interface can become cumbersome.
What is React?
React, developed by Facebook, is an open-source JavaScript library primarily used for building user interfaces, especially single-page applications that require a dynamic response from the user. React allows developers to create reusable UI components which manage their own state. These components can then be composed to create complex user interfaces.
A defining characteristic of React is the virtual DOM. Instead of directly manipulating the real DOM, React creates a lightweight copy. This approach speeds up updates and improves performance. For instance, when the state of a component changes, React efficiently updates only the components that need it, rather than re-rendering the entire application.
React's Role in Modern Web Development
React plays a pivotal role in modern web development. Developers are increasingly looking for solutions that enhance productivity and efficiency. With its focus on component-based architecture and virtual DOM, React reduces the burden of direct DOM manipulations, which are often slow and resource-intensive. This speed and efficiency are paramount in an age where user experience is king.
Moreover, the rise of mobile applications has propelled React to the forefront, particularly with the introduction of React Native. This framework allows developers to use the same codebase to create applications for both the web and mobile platforms. Therefore, understanding React is not just beneficial for web developers; it aids in expanding into mobile development as well.
Core Concepts of React
To fully appreciate the potential of React, one must grasp its core concepts:
- Components: React’s main building blocks. Each component encapsulates its own logic and rendering, making it easy to build and manage large applications.
- JSX (JavaScript XML): A syntax extension that allows mixing HTML with JavaScript. This makes the code more intuitive and easier to write.
- State and Props: State refers to the mutable data that affects how the component renders, while props are immutable data passed down from parent components.
- Lifecycle Methods: These methods allow developers to tap into specific points in a component's lifetime, enabling fine control over component behavior and performance.
Understanding these core concepts is essential for leveraging the full power of React in a CodeSignal integrated environment.
In summary, developing a deep understanding of React not only enhances one’s skillset as a developer but also greatly facilitates the integration process with tools like CodeSignal, paving the way for enriched user experiences and improved coding assessments.
Integrating CodeSignal with React
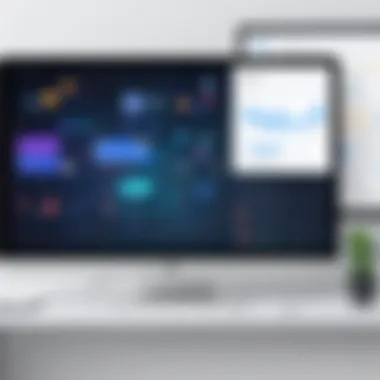
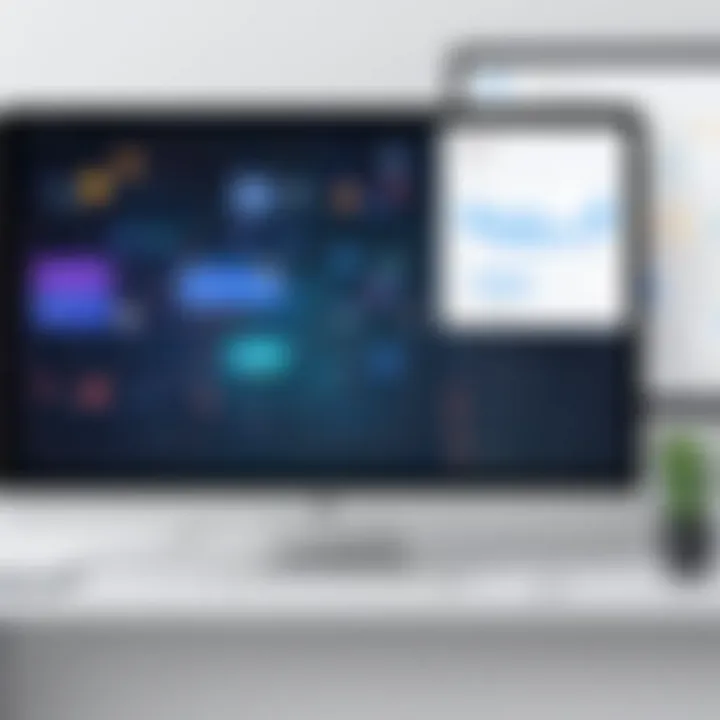
Integrating CodeSignal with React is a critical aspect for developers seeking to enhance their technical processes and application performance. This combination not only brings together a robust coding assessment platform with a thriving front-end library but also empowers organizations to evaluate developers' skills in a seamless manner. In today’s fast-paced software development landscape, leveraging tools that promote efficient coding practices is vital. When CodeSignal and React come into play, the synergy created helps in addressing key challenges of modern development.
The benefits of this integration are numerous. For one, speed and efficiency tend to boost as developers can evaluate their coding skills in real-time while working on user interfaces. Establishing a solid ground with CodeSignal helps to cultivate an environment of continuous learning and improvement among developers. Making use of structured coding assessments within React applications allows for quicker iterations with valuable feedback integrated into the workflow.
Setting Up the Environment
To kick things off, setting up the environment for integrating CodeSignal with React requires some foundational steps. First, ensure you have Node.js installed on your system; this is vital since React depends heavily on it. Once established, proceed to create your React application using Create React App, which facilitates setting up a new React project with sensible defaults.
After the initial setup, install the CodeSignal SDK. Following the documentation provided on CodeSignal's official website ensures you have all the necessary libraries in place to allow for proper integration. Configuring API keys and endpoints within your application is essential; it establishes a secure link between your app and the CodeSignal assessment platform.
Building the Basic Structure
With the environment set, the next logical step is to build the basic structure of your application. This means laying out your components and integrating CodeSignal functionalities within them. Start by creating a dedicated component, say , to handle assessments. This component will act as the main interface where users can select, start, and complete coding challenges.
Here's a simplified structure:
Ensure that the component has all necessary props and state management. Utilizing React hooks can be advantageous here, especially if you need to track assessment progress or results dynamically.
Using CodeSignal's API
Integrating CodeSignal's API involves a little bit of exploration into the robust capabilities it provides. The API allows you to manage various coding tasks, assess user performance, and obtain valuable insights. You can fetch coding challenges directly from CodeSignal, thereby enriching your app’s offering with real-world coding problems.
To call the API, ensure you have the right permissions and API tokens set up in your environment variables. Below is a sample code snippet demonstrating a simple API call to fetch coding tasks:
This code demonstrates fetching a list of coding tasks and displaying them. Make sure to handle errors and loading states appropriately to ensure a smooth user experience. Overall, employing CodeSignal’s API within your React application creates a dynamic and user-focused environment aimed at improving coding proficiency.
Benefits of Using CodeSignal in a React Application
Integrating CodeSignal within a React application brings several distinct advantages. This combination not only enhances the way developers assess and refine their coding skills but also optimizes the overall workflow. In this section, we’ll discuss three crucial benefits that stem from this integration: enhanced coding skills assessment, streamlined development workflow, and improved user engagement.
Enhanced Coding Skills Assessment
CodeSignal offers an evaluation framework that goes beyond the basics. With its emphasis on real-world problems, developers using CodeSignal within a React environment can measure their skills more accurately. This kind of assessment is crucial because it helps identify the strengths and weaknesses of a developer's coding proficiency.
Think of the misunderstandings during interviews when developers may not accurately showcase their abilities due purely to the format of typical coding interviews. CodeSignal can bridge that gap by simulating those real-life scenarios in an accessible platform that React developers are likely to appreciate.
Some of the notable features include:
- Customizable Assessment Tests: Tailor tests to match the requirements of specific projects or roles.
- Immediate Feedback: Developers receive quick insights on their performance, allowing for immediate improvements.
- Skill Tracking: Over time, users can monitor their growth and progress, which is vital for personal development.
Streamlined Development Workflow
The smooth integration of CodeSignal into a React application can significantly boost the development process. When developers test their skills directly within their working environment, tasks become less disjointed. It fosters a culture of continuous learning while reducing friction in the development lifecycle.
For instance, rather than waiting for separate assessments to be conducted, developers can run skills evaluations at any point. This immediacy enables team members to identify any gaps in knowledge and swiftly work to resolve them, increasing productivity and overall project success.
Consider these aspects that contribute to a streamlined workflow:
- Seamless Integration: CodeSignal fits right into existing React applications, minimizing hassle.
- Real-time Collaboration: Team members can collaborate on coding challenges, sharing insights and techniques.
- Documented Learning: Easily record and share learning outcomes within teams to nurture a learning environment.
User Engagement and Experience Improvement
Embedding CodeSignal in a React app also enhances user engagement, which is essential for retention and satisfaction. If users feel they are learning while using the platform, it promotes longer interactions. This engaging experience can translate into a powerful tool for businesses looking to attract and maintain talent.
Some key factors that improve user interaction are:
- Interactive Learning Modules: Gamifying challenges makes learning more appealing and less daunting.
- Analytics on Performance: Users can analyze their approaches and compare them against peers, igniting a competitive spirit that can enhance learning.
- Feedback Loops: Active feedback not only illuminates areas for improvement but also encourages users to continue pressing forward.
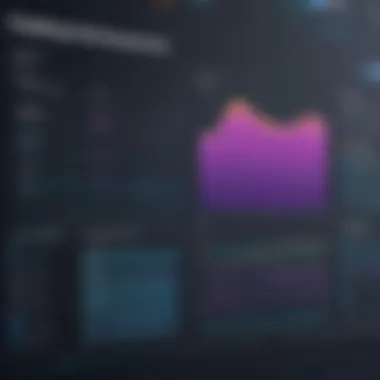
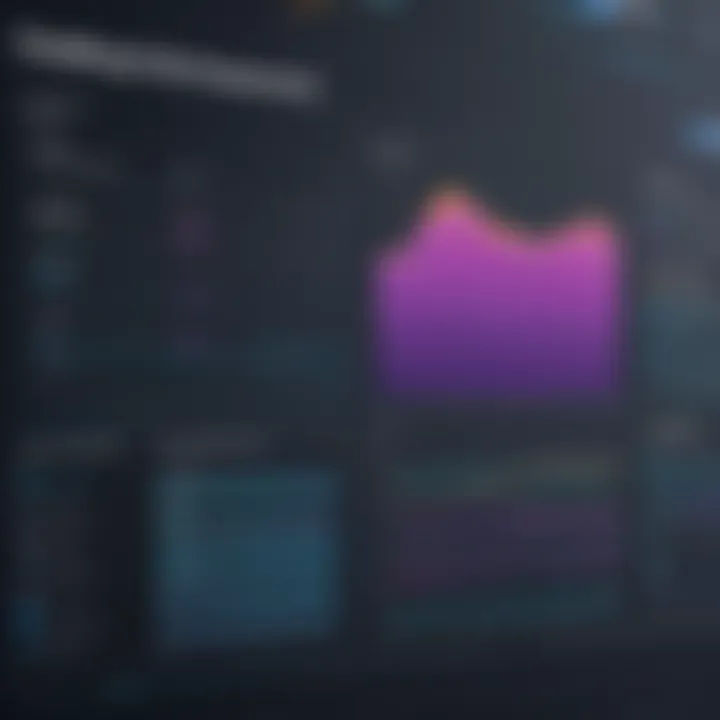
"User engagement results in better satisfaction and retention rates, which is crucial for any business targeting growth."
Challenges in Integration
When it comes to integrating CodeSignal with React, there are a few bumps in the road that developers need to navigate. These challenges can significantly impact the user experience and overall effectiveness of the integration, making it crucial to understand them thoroughly. Addressing these challenges isn't just about smooth sailing; it's about ensuring a well-functioning system that can genuinely enhance the coding experience.
Technical Obstacles
Every integration project faces technical hurdles, and the CodeSignal-React pairing is no exception. Issues can arise from, for instance, incompatibility between various versions of libraries. Developers often find themselves grappling with dependencies that may not play well together.
Moreover, the setup process can become a tangled web. Let’s say you are attempting to connect CodeSignal’s API with your React application. You must ensure that the proper endpoints are being called and that data formats match. Often, this becomes a trial-and-error process. API documentation, while usually good, can sometimes leave out crucial details that lead to confusion. An example of this might be improper handling of API responses, which can lead to frustrating debugging sessions.
User Adaptation Issues
Even when the technical side is neatly wrapped up, there's a second layer of challenges that kick in: user adaptation. Users, whether they are students learning to code or professionals brushing up on their skills, need time and guidance to adapt to new systems.
For instance, CodeSignal's unique assessment mechanics can throw off users who are already accustomed to different coding challenge platforms. This disconnect can lead to frustration, as they navigate unfamiliar functionalities. Training resources, guides, and some initial hand-holding can be vital for mitigating this issue, but they require effort and investment from the organization.
In many shops, time is of the essence; users want to get up to speed quickly. If the integration isn’t intuitive, you risk alienating them before they’ve had a chance to fully realize its benefits. Therefore, maintaining a support line or FAQ section becomes indispensable to tackle these hurdles effectively.
Maintaining Update Synchronization
Lastly, let’s not forget about keeping everything in sync when it comes to code updates. Both CodeSignal and React are evolving. Features are constantly rolling out, sometimes at an alarming pace. If your integration is running on outdated versions, you risk breaking functionality.
This challenge manifests when developers may not notice that an update in CodeSignal’s API has affected how data is being fetched in the React application. If these updates aren’t aligned, your integrated system could face downtime or critical failures. It’s like driving a car with an engine that doesn’t play nice with the latest software—sure, sometimes it’ll get you from A to B, but don’t be surprised if it stalls unexpectedly.
To mitigate these risks, implementing regular maintenance checks and a solid version control system is essential. You want to ensure compatibility with new features while also making sure that every user experience remains seamless. Developing a checklist for updates can simplify the routine process and help you keep tabs on necessary changes.
"If you are not anticipating change, you are not prepared for the future."
By embracing these challenges head-on, developers can set a strong groundwork that enables the effective combination of CodeSignal and React, paving the way for enhanced user experiences.
Evaluating Performance Metrics
When it comes to integrating CodeSignal with React, evaluating performance metrics becomes pivotal. Through this lens, stakeholders can gauge the efficiency and effectiveness of their coding assessments and overall application performance. Understanding how metrics can guide development efforts is essential, and this section will cover key aspects that would help you navigate this complex yet rewarding terrain.
Performance metrics are the lifeblood of any software application, especially in an environment focused on coding and testing. They help measure everything from user engagement and application speed to the overall accuracy of coding assessments. For IT professionals and organizations alike, these metrics are more than just numbers; they truly reflect user experiences and the real-world effectiveness of the tools in use.
Key Performance Indicators
In any systematic evaluation, identifying and tracking key performance indicators (KPIs) is the cornerstone. These indicators serve as quantifiable measures of product performance. Here are some crucial KPIs to keep an eye on when utilizing CodeSignal within a React application:
- Time to Complete Assessments: This metric shows how long users take to complete coding challenges. A shorter time might indicate that the challenges are well-suited to users’ skills, while long times could suggest that the questions are too complex or unclear.
- Pass Rates: Understanding the percentage of users who pass specific coding tests gives insights into the effectiveness of those assessments. A low passage rate may suggest the need for reevaluating the quality and difficulty of the tasks.
- User Engagement Rate: This measures how actively users interact with challenges over a given time frame. Higher engagement rates can indicate that users find the tasks interesting and relevant.
- Feedback Scores: Collecting user feedback on the challenging experience is invaluable. It provides an additional qualitative measure that highlights areas in need of improvement.
Assessing these KPIs regularly will offer a clearer picture of how well CodeSignal and React are working in tandem.
Data Analysis Tools
To effectively assess these performance metrics, one needs the right tools at their disposal. Data analysis tools not only help in collecting raw data but also provide frameworks for interpreting this data meaningfully:
- Google Analytics: Essential for tracking user interactions. It helps in analyzing the paths users take before and after completing coding challenges.
- Mixpanel: This tool goes beyond simple analytics; it provides cohort analysis which lets you see how specific segments of users behave over time.
- Tableau: A powerful data visualization tool that can turn this data into easy-to-understand dashboards. You can track KPIs and see trends over time, making insights actionable.
- Looker: Ideal for creating custom data models specific to your assessments, aiding in quickly identifying what works and what doesn’t.
By leveraging these tools, you can not only collect pertinent data but also derive insights that could fundamentally enhance how CodeSignal and React operate together.
In summary, evaluating performance metrics is critical. By focusing on key performance indicators and utilizing robust data analysis tools, you can make informed decisions that elevate user experiences while streamlining the development process.
Through careful evaluation and analysis, organizations can adapt their strategies, ensuring they meet user needs and remain competitive in the tech ecosystem.
User Feedback and Case Studies
User feedback and case studies play a pivotal role in understanding how well CodeSignal integrates with React applications. They provide insights that go beyond mere assumptions, revealing the actual performance in real-world conditions. These elements serve as valuable testimonies that can guide future users in their integration efforts, offering a glimpse into how various organizations have navigated challenges and harnessed the benefits presented by this combination. Dive into the importance of harnessing real-world insights and how they can inform decision-making for potential users.
Real-World Applications
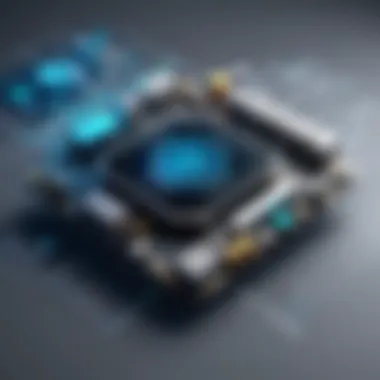
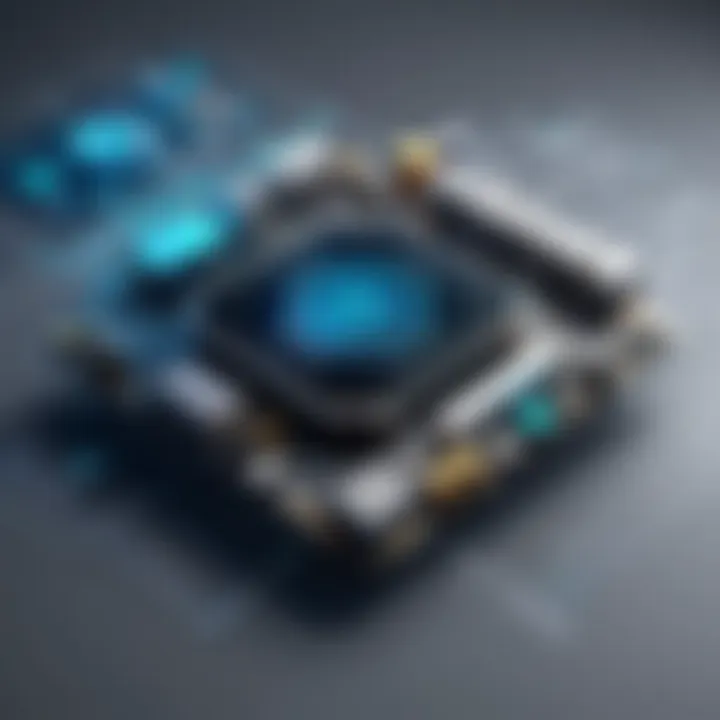
The practical application of CodeSignal within React projects can vary significantly across different industries. Many tech companies use this integration to enhance their hiring processes, enabling them to assess developer skills through simulation tasks directly within their applications.
For instance, consider a software firm that developed an assessment tool using React. They incorporated CodeSignal's APIs, allowing candidates to solve coding challenges in an interactive environment. By doing so, they not only provided a streamlined application experience but gathered data on candidates' performance in real-time. This approach made it easier for the HR team to identify top applicants, reducing the time taken in the selection process.
Here are some other specific examples of real-world applications:
- EdTech Platforms: Many educational platforms now incorporate CodeSignal for skill assessments integrated into their React-based interfaces, fostering an engaging learning atmosphere.
- Coding Bootcamps: These programs often utilize CodeSignal's tools within their curricula, enabling students to apply their skills immediately, ensuring better retention and understanding.
Success Stories
Success stories serve as powerful motivators for organizations looking to adopt CodeSignal with React. One notable instance is of a prominent technology consultancy that revamped their candidate assessment procedures. By incorporating CodeSignal into their React applications, they significantly improved their hiring metrics. The time to fill positions dropped dramatically, as the assessments were designed to mirror actual work scenarios.
Additionally, feedback from developers indicated a better understanding of the skills required for specific roles, thanks to the clarity CodeSignal assessments provided. This transformation led to a smoother onboarding process as new hires already possessed actionable insights into their roles.
"When we shifted to using CodeSignal within our React applications, it was like turning on the light in a dimly lit room. We could see clearly who was worth pursuing."
This consultancy's experience exemplifies how targeted assessments can lead to a more qualified talent pool.
Challenges Faced by Users
Despite the evident advantages, challenges in utilizing CodeSignal with React can arise. One major hurdle entails the steep learning curve associated with adjusting existing technologies and workflows to accommodate these tools. Many developers initially struggle with the integration of CodeSignal's APIs, often requiring additional training.
Consider a small startup which attempted this integration. They faced quite a few roadblocks, particularly with ensuring a seamless user experience. Initial feedback from candidates indicated confusion with the interface, resulting in a higher drop-off rate during assessments. Lessons learned from their experience included:
- Importance of User Testing: They quickly realized the need for testing their application more rigorously before launching.
- Iterative Improvements: Continuous feedback loops helped refine their integration, ensuring a user-friendly experience.
Moreover, maintaining an updated software environment that aligns with CodeSignal’s continuous advancements can be daunting for some organizations. It’s vital for teams to keep the lines of communication open, ensuring they maximize the value of this integration.
Future of CodeSignal and React Integration
The collaboration between CodeSignal and React is not just a fleeting trend in the realm of software development. This integration has the potential to evolve in ways that can significantly impact both developers and organizations alike. As we look ahead, it’s crucial to understand what this future might hold, the specific elements that will shape it, and the benefits that are likely to emerge.
This section delves into what lies ahead for the integration between CodeSignal and React. With the continuous evolution of technology, staying ahead of the curve is imperative. The future promises customization, enhanced learning tools, and an overall modular approach that can better serve developers and businesses.
Such integration opens doors to more targeted assessments, allowing developers to measure not just their coding skills but their problem-solving abilities in real-world scenarios.
Emerging Trends
As the tech landscape shifts, several notable trends are emerging around the integration of CodeSignal with React.
- Increased Personalization: Tools are now being designed to adapt to individual coding styles. Tailoring CodeSignal assessments to specific React projects could lead to more relevant skill evaluations.
- Focus on Collaborative Projects: There's a noticeable shift towards collaborative coding assessments. Developers are often required to work as a part of a team. Thus, combined platforms could provide assessments that simulate team environments, helping companies gauge collaboration skills.
- AI-Powered Insights: Artificial Intelligence is becoming a cornerstone in software development. CodeSignal is likely to incorporate more AI-driven insights tailored to React, helping developers identify their strengths and weaknesses more accurately.
These trends indicate that the integration between CodeSignal and React is not a static solution but a dynamic one that will adapt to the needs of the community.
Potential Developments
Looking further into the horizon, several potential developments could redefine the integration between CodeSignal and React, with far-reaching implications.
- Advanced APIs: Future iterations may include more sophisticated APIs that allow developers greater freedom in tailoring CodeSignal's functionality directly into their React applications. This could mean less friction when setting up assessments or scoring systems, promoting a seamless experience.
- Comprehensive Skillset Training: The merging of learning pathways from CodeSignal and practical coding experiences within React could pave the way for highly specialized training tools, addressing both hard and soft skills necessary for modern developers.
- Broader Ecosystem Partnerships: As companies look to bolster productivity, partnerships with other platforms can emerge. Imagine integrating CodeSignal assessments into project management tools like JIRA, resulting in a multi-faceted development environment.
These developments suggest that as CodeSignal and React continue to evolve in tandem, the ecosystem surrounding them could become richer and more diverse. The focus will likely extend beyond mere assessment to encompass an all-encompassing growth model for developers.
"The next phase in the integration of CodeSignal and React will not just focus on skill assessment but on creating an environment for continuous learning and development."
As we step into this promising future, the desire for innovation and improved user experience will drive the integration further. Whether you’re an IT professional looking to sharpen skills or a small business seeking an edge in the competitive market, understanding these trends and potential developments will position you advantageously.
Ending
In summing up our exploration of integrating CodeSignal with React, it's clear that this topic holds considerable weight for professionals engaged in software development. The integration facilitates robust coding skill assessments while allowing developers to create interactive user interfaces. This duality not only enhances the application’s usability but also ensures that the quality of code is maintained throughout the development lifecycle.
Being able to effectively evaluate coding skills can significantly influence hiring and development processes. In a landscape where tech roles are ever-evolving, CodeSignal offers businesses a way to tailor assessments specifically to their tech stack while React provides a flexible framework for building engaging applications. With both tools working hand-in-hand, teams can streamline their workflows, resulting in efficiency and a deeper understanding of coding practices.
Moreover, it's essential to recognize the challenges that may arise during integration, as they are part and parcel of the development journey. Addressing these challenges head-on—whether they involve technical obstacles or user adaptation—can lead to stronger solutions and an increased overall satisfaction among stakeholders.
Recap of Key Points
- CodeSignal enables skill assessment which is critical for recruitment and team capabilities.
- React is versatile, allowing for beautiful yet powerful user interfaces, making it a go-to choice for developers.
- Integration of CodeSignal with React enhances the development process, leading to faster delivery times and fewer errors.
- Addressing integration challenges requires a proactive approach to ensure smooth implementation.
Final Thoughts on Integration
The future outlook for integrating CodeSignal with React is promising. As both technologies continue to evolve, staying informed on emerging trends and potential developments can provide a strategic advantage. It is vital for professionals in the field to monitor advancements in these platforms, as they will inevitably lead to new methodologies that can greatly benefit software development practices.