Understanding the Java Framework: Architecture and Evolution
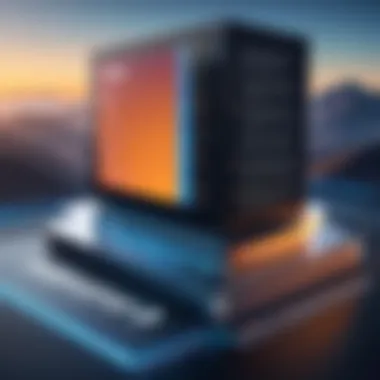
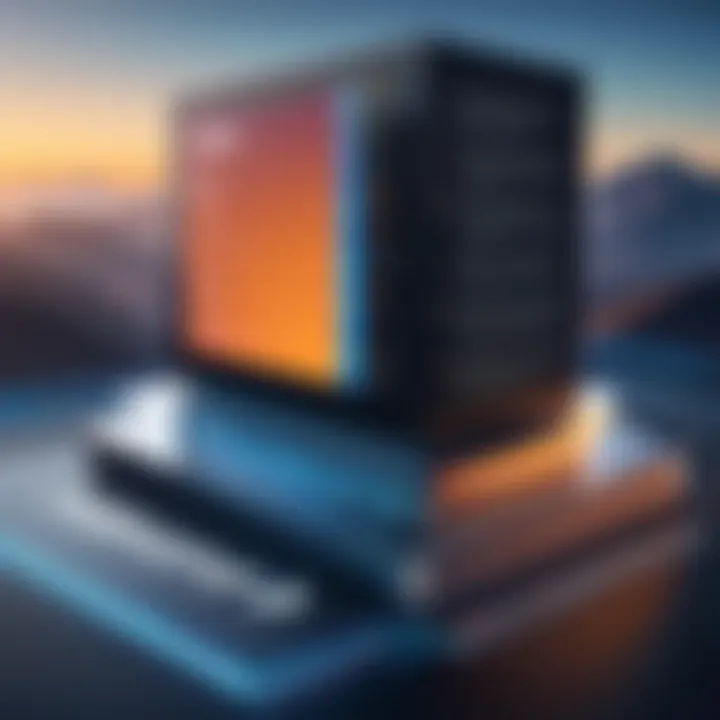
Intro
Java is not merely a programming language; it represents a versatile framework that has significantly transformed software development. Understanding how Java operates, its architecture, and its prominent characteristics is essential for developers. This exploration will delve into the multifaceted nature of Java, thereby shedding light on its applications in various environments.
Overview of Software
Description of Software
The Java framework, grounded in the principles of object-oriented programming, offers a comprehensive ecosystem for creating robust applications. It encompasses a wide range of libraries, tools, and APIs that simplify the development process while enabling high performance and scalability. Used globally, it facilitates the creation of web applications, mobile apps, and enterprise-level systems.
Key Features
Some key features of the Java framework include:
- Platform Independence: Java follows the principle of "write once, run anywhere." This means that Java applications can run on any device equipped with a Java Virtual Machine (JVM), enhancing compatibility across platforms.
- Rich API: Java provides a rich set of libraries and APIs that streamline development. These resources cover various functionalities such as networking, data manipulation, and graphical user interfaces.
- Strong Community Support: With a vast community of developers, resources for learning and problem-solving are readily available. This fosters an environment of collaboration and innovation.
- Security: Java offers robust security features, making it a popular choice for enterprise applications. The architecture includes an application sandbox and bytecode verification for enhanced safety.
- Multithreading: This feature allows concurrent execution of programs, ensuring that multiple operations can happen simultaneously, improving application performance and responsiveness.
"Javaβs design promotes software portability, making it a quintessential choice for developers exploring cross-platform solutions."
Software Comparison
Comparison with Similar Software
When comparing Java with other frameworks like Python's Django or JavaScript's Node.js, some distinctions emerge. Python, for example, offers simplicity and ease of learning, making it suitable for rapid development. Node.js excels in handling asynchronous tasks efficiently; however, it may lack the security features and robustness that Java provides.
Advantages and Disadvantages
Advantages
- Portability: Java applications can run on any operating system, promoting flexibility.
- Performance: Through Just-In-Time compilation, Java can achieve high performance.
- Strong Typing: Reduces runtime errors through enforced type-checking during compile time.
Disadvantages
- Memory Consumption: Java can be less efficient in memory usage compared to some other languages.
- Verbosity: Developers may find Java code more verbose than necessary, which can impact readability.
- Slower than Native Languages: For certain applications requiring maximum performance, languages like C++ may be preferred.
In summary, the Java framework remains a pivotal element of software development, demonstrating remarkable capabilities and adaptations over time. As it evolves, understanding its fundamental principles and contemporary applicability becomes crucial for developers aiming to leverage its full potential.
Prelude to Java Frameworks
Java frameworks are an essential element of modern software development, providing a structured way to build applications efficiently. As software needs grow increasingly complex, frameworks play a vital role in facilitating the development process. By offering pre-built modules, libraries, and tools, these frameworks allow developers to focus on writing business logic rather than dealing with repetitive tasks.
Frameworks can greatly reduce development time and improve code quality. They promote best practices, encourage modular design, and help in maintaining consistency across various projects. Without frameworks, development can become chaotic, leading to code that is difficult to maintain or scale.
In this article, we will explore the various types of Java frameworks available, their core components, and their significance in the industry. Understanding these frameworks is not just important for individual developers but equally crucial for organizations aiming to optimize their workflow and enhance productivity.
Definition of Java Framework
A Java framework is a platform that provides developers with a foundation to build applications in the Java programming language. It consists of a collection of libraries, APIs, and conventions that serve as a guide to develop software applications more effectively. Frameworks define a structural skeleton which developers can use to assemble their code. Popular examples include Spring, Hibernate, and JavaServer Faces (JSF).
The primary goal of a framework is to simplify the development process. By utilizing a framework, developers can avoid the boilerplate code that often comes with application development. Furthermore, frameworks often encapsulate best practices, making it easier to apply them consistently across projects.
Importance of Frameworks in Java Development
Frameworks are an integral part of Java development for several reasons:
- Enhanced Productivity: By providing ready-to-use components, frameworks allow developers to complete tasks faster.
- Code Consistency: Frameworks promote standardized coding practices which lead to better readability and maintainability.
- Community Support: Popular frameworks like Spring and Hibernate come with robust community support, offering extensive documentation, forums, and resources.
- Easier Testing: Many frameworks include built-in tools and practices that facilitate unit testing and integration testing, which is key in modern development workflows.
"Using a well-designed framework can cut development time in half while ensuring higher quality output."
Historical Context
Understanding the historical context of Java frameworks is essential for grasping their importance in modern software development. This segment highlights how Java has evolved over time and how this evolution has shaped the frameworks we rely on today. Recognizing this history can offer insights into current best practices and future trends, informing developers about the decisions that have led to the frameworks we use.
Evolution of Java
Java was introduced by Sun Microsystems in 1995. From its inception, it focused on write-once, run-anywhere capabilities, which made it uniquely attractive for developers. The language quickly gained popularity due to its strong object-oriented features, a comprehensive set of APIs, and a significant community for support and development questions.
Over the years, Java underwent various updates. Key versions introduced new features. For instance, Java 2 introduced the Swing graphical API, and Java 5 brought important changes, including generics and annotations. Each evolution enhanced Javaβs flexibility and power. The transition to a more modern development approach, especially with Java 8, marked a significant turning point. Lambda expressions and the Stream API enabled more functional programming paradigms, making code more efficient and easier to read.
Development of Java Frameworks
The growth of Java frameworks began in response to the increasing complexity of application development. Frameworks provide structures that simplify coding, making it easier to build robust applications. Several frameworks emerged in the late 1990s and early 2000s, each designed to address specific development challenges.
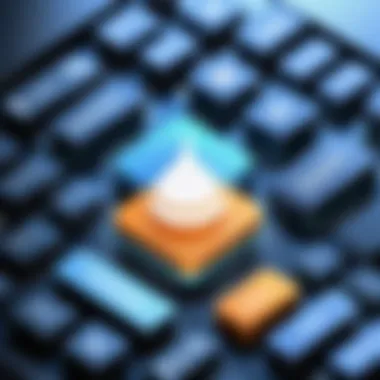
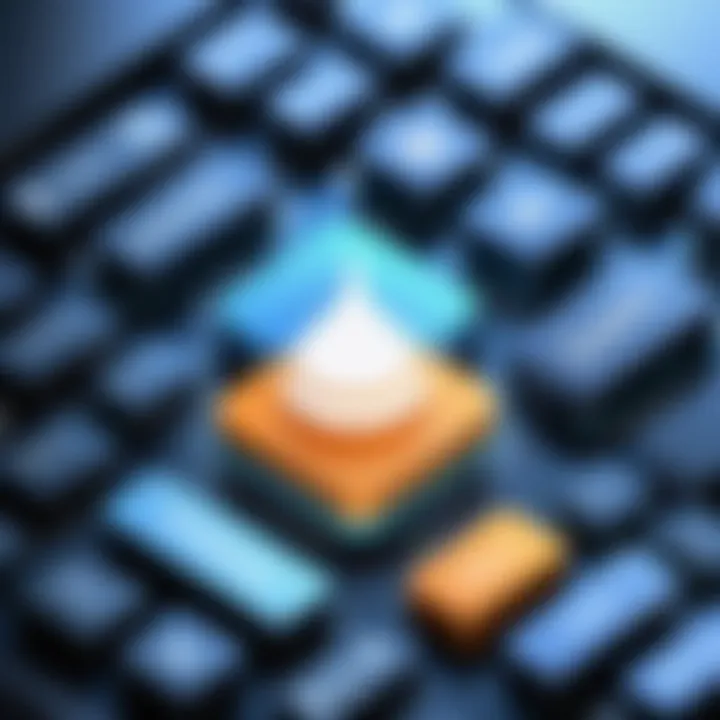
The first notable framework, Struts, introduced in 2000, provided a model-view-controller architecture that allowed developers to separate business logic from presentation. This set the standard for future frameworks.
Following Struts, Spring Framework appeared in 2003. Spring introduced dependency injection, promoting loose coupling in application design. This innovation has greatly influenced how modern applications are structured.
Today, the Java framework ecosystem includes a variety of optionsβsuch as Hibernate for data persistence and Java Server Faces for user interfacesβeach of which plays a role in the comprehensive Java development landscape. These frameworks are not only popular but are also vital to the efficiency and structure of Java-based applications.
Overall, the historical context of Java and its frameworks is a testament to the adaptive nature of the language and its community. It reflects ongoing responses to developer needs and the pursuit of improved practices in software development. This historical lens deepens our understanding of why certain frameworks are preferred and how they can be effectively utilized.
Core Components of Java Frameworks
Java frameworks serve as a foundation for building applications efficiently. The core components of these frameworks are critical in understanding how they simplify development processes and enhance productivity. These components include the architecture, libraries, tools, and various support mechanisms that all work together to provide a comprehensive development environment.
Within the Java ecosystem, these core components dictate how developers approach software development. They influence everything from project structure to coding practices. Recognizing the significance of core components helps developers understand what frameworks best align with their needs, ultimately leading to more effective and robust applications.
Architecture Overview
The architecture of a Java framework defines its structure and interactions between various components. Most Java frameworks are layered constructions, which separate concerns and facilitate maintenance and scalability. In general, a typical architecture includes the following layers:
- Presentation Layer: This layer handles user interaction, creating the user interface and managing the user experience.
- Business Logic Layer: Here, the application processes data and implements core functionalities. This is where the majority of custom code resides.
- Data Access Layer: This layer manages data persistence and database interactions. It connects business logic with data storage solutions, such as relational databases.
Frameworks like Spring or Hibernate leverage these architectural layers to promote best practices in software design. This division not only promotes clear responsibilities but also allows teams to work independently on different aspects of the application.
"A well-defined architecture enables a smoother development process, allowing teams to encounter fewer hurdles in collaboration."
Understanding these layers helps in selecting the appropriate tools and libraries for a given project. It also guides the developers in adhering to coding standards and principles pertinent to their chosen framework.
Key Libraries and Tools
Key libraries and tools are fundamental to the Java framework experience. They extend the capabilities of the framework, support repetitive tasks, and streamline complex processes. Some pivotal libraries and tools developers often utilize include:
- Spring Framework: Provides comprehensive infrastructure support for developing Java applications. It simplifies the use of Java through dependency injection, aspect-oriented programming, and more.
- Hibernate: This library streamlines data operations and object-relational mapping (ORM), handling database connectivity without cluttering code with SQL queries.
- Apache Maven: A powerful project management tool that executes many tasks such as building, testing, and deploying applications, simplifying the overall workflow.
- JUnit: An essential library for unit testing, ensuring code correctness and adherence to requirements.
The selection of appropriate libraries and tools can greatly expedite development. They offer reusable code, saving time and effort. Moreover, these libraries often come with community support and extensive documentation, bridging knowledge gaps for developers, especially those who are new to the Java ecosystem.
Spring Framework
The Spring Framework stands as a monumental pillar in Java development, offering a robust platform that addresses the complex requirements of enterprise applications. It emphasizes the importance of loose coupling, making it easier for developers to manage large codebases. The architectural pattern provided by Spring enables better separation of concerns, which is vital in developing scalable applications. In this section, we will explore the foundational aspects, significant features, and the simplifications that Spring Boot introduces into the development process.
Foreword to Spring
The Spring Framework originated in the early 2000s, spearheaded by Rod Johnson. It was designed to simplify Java enterprise application development by addressing various challenges inherent in heavyweight Java EE infrastructure. Spring's comprehensive approach integrates core capabilities with a robust support system for building applications. At its heart, Spring centers around the concept of inversion of control (IoC), which allows developers to focus more on application logic rather than encounter boilerplate code.
In addition to IoC, Spring offers an extensive ecosystem that caters to various needs, including web applications, security, and data access. As a modular framework, it allows developers to choose specific components tailored for their projects, enhancing flexibility and efficiency.
Features and Benefits of Spring Framework
Spring Framework is renowned for its numerous features that simplify development while enhancing performance. Some of the most notable include:
- Dependency Injection: This is central to Spring's IoC container. It allows objects to be created with their dependencies provided by the container, reducing coupling and increasing testability.
- Aspect-Oriented Programming (AOP): Spring supports AOP, enabling the separation of cross-cutting concerns, such as logging and transaction management, improving code maintainability.
- Transaction Management: Spring offers a consistent programming model for transaction management, which simplifies working with different transactional APIs.
- Integration with Other Frameworks: Spring seamlessly integrates with various frameworks, including Hibernate, JPA, and JSF, providing developers with the flexibility to use technologies that best fit their projects.
- Web Capabilities: Spring MVC is a powerful component for building web applications. It caters to the design of RESTful services and manages UI elements effectively.
The benefits of using Spring extend beyond features; they encompass increased productivity, reduced boilerplate configuration, and customizability that aligns with specific business needs.
Spring Boot: Simplifying Development
Spring Boot emerges as an enhancement of the Spring Framework. Its primary goal is to minimize the complexity of configuring Spring applications, enabling developers to focus on building rather than setting up. With Spring Boot, you can get started quickly, deploying applications with minimal fuss.
Key aspects of Spring Boot include:
- Convention over Configuration: Spring Boot uses defaults to simplify setup. Configuration is automatic, removing much of the burden from developers.
- Embedded Servers: Spring Boot eliminates the need for external web servers by incorporating embedded servers like Tomcat or Jetty, streamlining deployment and testing.
- Microservices Ready: Its design aligns perfectly with microservices architecture, facilitating the building of small, independent applications.
- Production Ready: Out-of-the-box metrics, health checks, and externalized configuration help in deploying applications that are stable and robust without significant extra work.
Spring Boot transforms Java development efficiency. By removing traditional complexities, it empowers developers to create reliable applications swiftly. In a world where speed and adaptability are essential, Spring Boot is a crucial tool for any Java developer.
"Spring Framework embodies simplicity and flexibility, redefining enterprise application development."
Overall, the Spring Framework is not just another tool but a comprehensive ecosystem poised to meet the evolving demands of modern software development.
Hibernate and JPA
Java frameworks have revolutionized the way developers approach persistence in applications. Hibernate and Java Persistence API (JPA) play critical roles in this aspect. They simplify the process of interacting with databases, handling both data storage and retrieval with efficiency. The use of Hibernate and JPA provides significant benefits that contribute to better application performance and developer productivity.
Understanding Hibernate
Hibernate is an open-source Object-Relational Mapping (ORM) framework for Java. It allows developers to map Java classes to database tables, significantly simplifying data manipulation. With Hibernate, the concern of managing the SQL queries is reduced as it translates object-oriented Java programming into relational database interactions seamlessly. One notable feature is its capability to handle multiple database dialects.
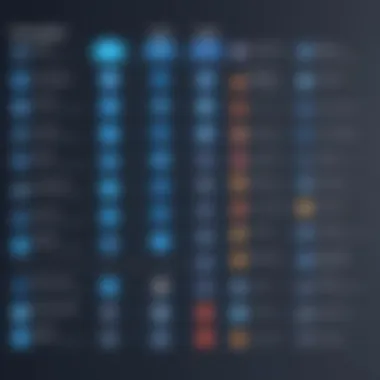
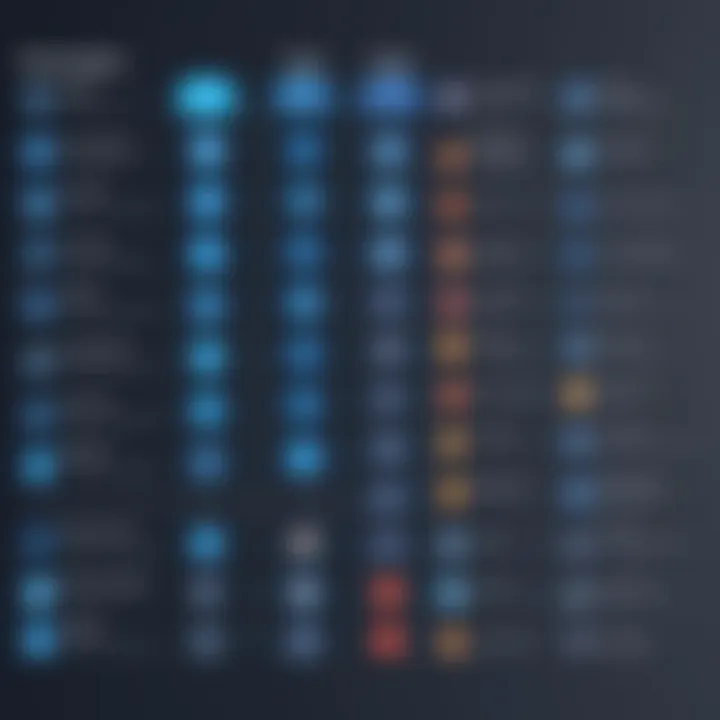
Key points about Hibernate include:
- Automatic SQL generation: Hibernate generates SQL for you based on your mapping, reducing coding overhead.
- Caching mechanisms: It supports first-level and second-level caching, which enhances performance by limiting database access.
- Lazy loading: Hibernate allows deferring the loading of data until it is actually needed, promoting efficiency in resource utilization.
Java Persistence API Overview
Java Persistence API (JPA) is an essential part of the Java EE standard. It provides a set of guidelines for ORM in Java applications. JPA relies on various implementations, and Hibernate is one of the most popular among them. JPA standardizes the way developers handle database operations, enabling more straightforward interaction with relational databases.
Key aspects of JPA are:
- Entity Management: Defines how data objects map to database tables.
- Query Language: JPA defines a query language called JPQL, which is similar to SQL but focuses on entity objects instead of tables.
- Transaction Management: It integrates with the Java Transaction API, making it easier to manage database transactions in applications.
Integration of Hibernate with Spring
Integrating Hibernate with Spring is common practice among Java developers. Spring provides various features that simplify the use of Hibernate within its ecosystem. The integration focuses on leveraging Springβs configuration options and transaction management capabilities.
Some benefits of this integration include:
- Dependency Injection: Spring allows for easy management of Hibernate sessions, promoting clean code practices.
- Declarative Transactions: Developers can manage transactions without boilerplate code, enhancing maintainability.
- Event-driven Programming: Utilizing Springβs event-driven approach allows for reactive and responsive applications.
By integrating Hibernate into Spring frameworks, developers can create robust applications with minimal configuration overhead.
Java Server Faces (JSF)
Java Server Faces (JSF) plays a pivotal role in the Java ecosystem, especially when it comes to building web applications. This framework offers a standardized approach to developing user interfaces for Java EE applications. As web applications increasingly demand robust and dynamic interfaces, understanding JSF becomes essential for any developer working within this space.
Intro to JSF
JSF is a Java specification for building component-based user interfaces for web applications. Introduced by Oracle, it simplifies the development integration of web-based interfaces by providing a set of reusable UI components. These components can be easily manipulated and configured, which allows developers to focus on the functionality rather than the intricacies of HTML, CSS, and JavaScript. One important aspect of JSF is its ease of binding UI components directly to backend data models. This makes it an effective tool for developers aiming to create interactive applications without deep diving into the finer details of frontend coding.
JSF operates on the principle of component-based architecture, allowing developers to create reusable UI elements, which can significantly reduce redundancy in web applications. The use of POJOs (Plain Old Java Objects) within this framework enhances its flexibility and integration in Java technologies.
Benefits of Using JSF
There are multiple benefits to employing JSF in web application development:
- Component Reusability: Developers can create and use a library of UI components, which drastically reduces development time.
- Ease of Maintenance: A component-based approach makes it easier to update or modify UI elements without affecting the overall application structure.
- Rich Ecosystem: JSF has a rich set of libraries and tools available, such as PrimeFaces and RichFaces, which can enhance user interface components further.
- Ajax Support: It includes built-in support for Ajax, enabling partial page rendering, which improves the user experience by reducing load times and enhancing interactivity.
- Standardization: Being a part of the Java EE stack, JSF offers a standardized way of performing development that aligns with Java's principles. This standardization leads to better collaboration in teams working on large projects.
JSF is not just about simplifying web application development; it sets the stage for scalable, maintainable, and user-friendly applications in enterprise environments.
While JSF offers several advantages, it is essential to consider the learning curve associated with its component-based architecture. Developers may need some time to fully grasp the framework's capabilities, especially if they are accustomed to traditional HTML and JSP-based approaches.
In summary, Java Server Faces stands out as a robust framework that addresses various challenges in building modern web applications. Its focus on component-based development, along with rich features and support for latest technologies, makes it a valuable tool in any developer's arsenal.
Apache Struts
Apache Struts is a significant framework within the Java ecosystem. It facilitates the development of web applications by providing a structured approach that encourages developers to follow best practices. The framework is particularly valuable for managing the complexities of enterprise-level applications. With its emphasis on MVC (Model-View-Controller) architecture, it enhances the separation of concerns, leading to better maintainability and scalability of applications. This section delves into the intricacies of Apache Struts, examining its core features and real-world applications.
Overview of Apache Struts
Apache Struts, initiated in 2000, has been instrumental in shaping Java web development. It is based on the MVC pattern, guiding developers to structure applications in a methodical way. In this framework, the Model handles the data, the View displays the interface, and the Controller processes input. This triadic separation simplifies the development and modification of complex systems.
Struts operates on a configuration-based style, allowing developers to define their application's behavior through XML. This approach provides a centralized way to manage various aspects, such as action mappings, result pages, and even forms. Moreover, Apache Struts has a vibrant community and extensive documentation, which can be crucial for developers facing issues or looking for guidance.
Key Features and Applications
One of the most notable features of Apache Struts is its extensibility. Developers can integrate additional libraries to enhance functionality, which promotes agility in project development. The framework offers various components, making it versatile for different types of applications.
Key features of Apache Struts include:
- MVC Architecture: Encourages organized code and encourages collaboration among different developer roles.
- Tag Libraries: Simplifies the creation of user interfaces by enabling easy integration of complex HTML and CSS functionality.
- Internationalization Support: Facilitates the creation of multilingual applications, catering to a global audience.
- Validations: Built-in validation framework ensures that data processing adheres to defined rules.
Applications of Apache Struts can be seen across several domains. Many enterprise applications rely on its structure for efficient resource management. Websites that require significant data interaction benefit from its solid underpinnings, allowing for quick updates and expansion.
To summarize, Apache Struts remains relevant because it champions the principles of modularity and separation of concerns, both of which are essential in modern web application development. By adhering to these principles, developers ensure a more sustainable and manageable codebase.
Java Frameworks Comparison
The comparison of Java frameworks holds significant relevance in the context of this article. With numerous frameworks available today, each offering unique features and functionalities, developers face the challenge of selecting the most suitable framework for their specific needs. Understanding the variations between frameworks can lead to informed decisions that enhance productivity and code quality. This section will explore the strengths and weaknesses of various frameworks, as well as provide practical use cases that illustrate their applications in real-world scenarios.
Strengths and Weaknesses
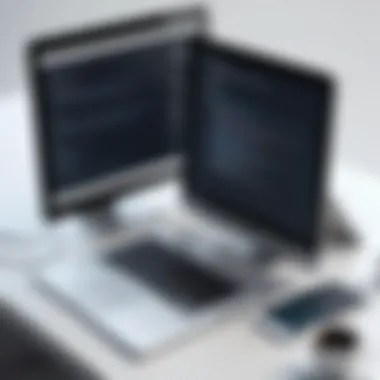
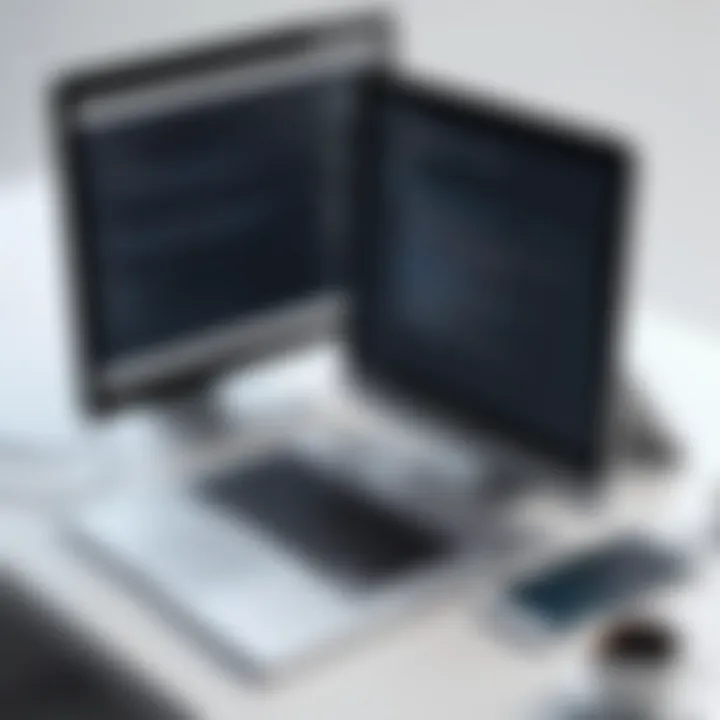
When choosing a Java framework, it is crucial to analyze both its strengths and weaknesses. This helps developers understand how well a framework aligns with their project requirements.
- Strengths
- Weaknesses
- Community Support: Popular frameworks such as Spring and Hibernate have vast communities that contribute to extensive documentation and resources.
- Flexibility: Frameworks like Spring Boot allow customizability, making them adaptable to different project sizes and complexities.
- Integration: Many frameworks offer seamless integration with modern development tools, databases, and middleware.
- Performance Optimization: Some frameworks are optimized for performance, providing faster processing and lower resource consumption.
- Learning Curve: Frameworks like JavaServer Faces can present a steep learning curve for newcomers.
- Overhead: Certain frameworks may introduce unnecessary complexity leading to slower performance in smaller applications.
- Dependency Management: Some frameworks require extensive configuration, which could complicate deployment processes.
Due to these considerations, developers must weigh these strengths and weaknesses carefully to decide which framework aligns best with their project goals.
Use Cases for Different Frameworks
Selecting the appropriate Java framework also involves assessing practical use cases. Different frameworks serve specific purposes in software development, and knowing when to apply them can significantly affect the outcome of a project.
- Spring Framework
The Spring Framework is ideal for building enterprise-level applications, especially those requiring complex business logic. Its ability to manage dependencies via inversion of control is particularly useful for large applications. - Hibernate
Perfect for applications that require robust data handling, Hibernateβs object-relational mapping capability simplifies database interactions while providing a powerful query language through Hibernate Query Language (HQL). - JavaServer Faces (JSF)
When developing user interfaces for web applications, JSF is effective. It streamlines the building of component-based user interfaces, making it suitable for applications that focus heavily on user experience. - Apache Struts
Struts is best suited for applications needing a well-structured framework for building MVC-based web applications. Its clear separation of concerns makes it easy to maintain and scale.
Best Practices in Using Java Frameworks
Java frameworks provide a structured approach to software development, guiding developers through the complexities of building applications. Employing best practices significantly enhances productivity, quality, and maintainability. This section explores critical elements and considerations when using Java frameworks, ensuring the effective application of these tools.
Code Structure and Organization
Maintaining a well-organized code structure is essential in Java framework development. It ensures that the code is manageable and understandable, which is vital when teams scale or projects evolve. Here are key strategies to enhance code structure:
- Segregate Functional Modules: Break the application into smaller, independent modules. This separation allows for easier debugging and testing.
- Embrace Layered Architecture: Use layered architecture to define clear boundaries between the data layer, business layer, and presentation layer. Each layer should have specific responsibilities, improving clarity and reducing interdependencies.
- Follow Naming Conventions: Consistent naming conventions give meaning to classes, methods, and variables, making the code self-explanatory. Use standard Java naming conventions to ensure familiarity for all developers.
- Comment Strategically: While excessive comment burden the code, selective use of comments can clarify complex logic or non-obvious design decisions. Focus on adding comments where the rationale is not immediately clear to someone else reading the code.
These practices will not only streamline the development process but also facilitate collaboration among team members. As mention earlier, this can significantly reduce onboarding time for new developers.
Utilizing Annotations Effectively
Annotations in Java frameworks play a crucial role in simplifying configuration and enhancing readability. Understanding how to use annotations effectively can yield numerous benefits:
- Reduce Boilerplate Code: Annotations help minimize repetitive coding constructs, allowing developers to focus on business logic. For instance, using Spring's annotation eliminates the need for explicit dependency injection code.
- Improve Readability: Annotations clarify the role of certain classes or methods, making the intentions behind the code more apparent. For example, clearly indicates that a method involves a database transaction, guiding other developers about the methodβs behavior.
- Centralized Configuration: In frameworks like Spring, annotations enable configuration to be centralized, reducing the need for external XML or property files. This centralization simplifies application setup and adjustments in configuration parameters.
- Support for Custom Annotations: Developers can create custom annotations tailored to specific needs, enabling a more intuitive way to enforce certain behaviors or validate data across the application.
When used thoughtfully, annotations enhance the development experience and overall application performance. It is important to ensure they are applied consistently and meaningfully throughout the codebase.
Effective use of code structure and annotations not only broadens maintainability but also fosters a collaborative environment that encourages best coding practices in Java framework development.
Future Trends in Java Framework Development
Understanding the future of Java frameworks is crucial for developers and businesses alike. As technology advances, the demand for more efficient, scalable, and flexible solutions grows. Developers need to stay informed on emerging trends that can shape their projects and their development strategies. This section will delve into significant elements influencing the future of Java frameworks, the potential benefits for organizations, and crucial considerations that must be addressed.
Emerging Technologies
The landscape of Java frameworks will be significantly influenced by new technologies. Here are some trends to keep an eye on:
- Microservices Architecture: This approach encourages the development of applications as a collection of small, independent services. Java frameworks like Spring Boot support this model, improving agility and scalability.
- Serverless Computing: Frameworks will increasingly support serverless architectures, reducing operational overhead. With AWS Lambda and Azure Functions, developers can focus on writing code without worrying about server management.
- AI and Machine Learning: Integration of AI capabilities within Java frameworks can enhance application intelligence. Libraries tailored for machine learning can facilitate complex data analysis and real-time decision-making.
- Reactive Programming: This paradigm allows developers to build applications that are responsive, resilient, and scalable. Java frameworks like Spring WebFlux are focusing on reactive programming to manage asynchronous data streams effectively.
Adopting these technologies can significantly improve project outcomes. The key is to determine which trends align best with specific project needs and goals.
Predictions for Java Frameworks
Looking ahead, several predictions can be made concerning the evolution of Java frameworks:
- Continued Growth of Spring Framework: Spring is expected to remain a dominant player due to its versatility and broad adoption across industries. Enhancements to Spring Boot and Spring Cloud are likely to make microservices development even more streamlined.
- Increased Focus on Security: As cyber threats grow, frameworks will need to prioritize security features. Java frameworks may integrate advanced security measures by default, simplifying compliance and protecting user data.
- Enhancements in Performance: The demand for faster applications will drive frameworks to innovate further. This may lead to the creation of new tools that optimize performance at every level of the application stack.
- Better Developer Experience: The focus on developer productivity will lead to improved tooling, documentation, and community support. This will help reduce the learning curve for frameworks and enable developers to be more effective.
- Community Engagement and Open Source Growth: The open-source nature of many Java frameworks will support continuous evolution. Community contributions will remain a vital aspect of driving future developments.
"The future often holds unexpected paths. Staying current with trends will ensure relevance in a rapidly changing tech landscape."
In summary, the direction of Java frameworks will be heavily influenced by emerging technologies and the evolving needs of developers and businesses. Embracing these changes can drive innovation and efficiency in software development.
Closure
The conclusion of this article serves as a vital encapsulation of the extensive exploration we have conducted into the realm of Java frameworks. Throughout this journey, we have unpacked the multifaceted nature of these frameworks, analyzing their architecture, components, and historical context. Understanding the role and functionality of Java frameworks is more than just academic; it directly correlates to the efficiency and effectiveness of modern software development.
Recap of Key Points
In our examination, we have highlighted several key aspects of Java frameworks:
- Definition and Importance: Java frameworks provide the necessary structure for building applications. They enhance productivity and provide organized code which simplifies maintenance and scalability.
- Historical Evolution: We traced the evolution of Java and its frameworks, noting how they adapted to meet emerging technology needs.
- Framework Implementation: We discussed popular frameworks like Spring, Hibernate, and Apache Struts, elucidating their unique features and applications in real-world scenarios.
- Best Practices: Emphasis was placed on the importance of adhering to best practices, which guide developers in maintaining a clean codebase and optimizing performance.
- Future Trends: Finally, we highlighted some future trends that may shape the landscape of Java frameworks, urging professionals to stay ahead in this fast-evolving field.
These points collectively outline the indispensable role Java frameworks play in the software development ecosystem.
Final Thoughts on Java Frameworks
As the world of technology continues to develop, the role of Java frameworks will likely expand. These frameworks not only streamline development but also foster innovation within applications. For IT professionals and software developers, staying informed about these frameworks enables them to leverage new features and architectures that can dramatically affect project outcomes.
"Java frameworks are more than just tools; they are partners in the journey of software development, offering structure, clarity, and efficiency."
By engaging with this article, professionals are better equipped to approach their projects with a broader perspective and a deeper understanding of Java frameworks, ultimately leading to better outcomes.